SpecFlow is a testing framework that supports Behaviour Driven Development (BDD). It lets us define application behavior in plain meaningful English text using a simple grammar defined by a language called Gherkin.
Use SpecFlow to define, manage and automatically execute human-readable acceptance tests in .NET projects. Writing easily understandable tests is a cornerstone of the BDD paradigm and also helps build up a living documentation of your system.
SpecFlow integrates with Visual Studio, but can be also used from the command line (e.g. on a build server). SpecFlow supports popular testing frameworks: MSTest v2, NUnit 3 and xUnit 2.
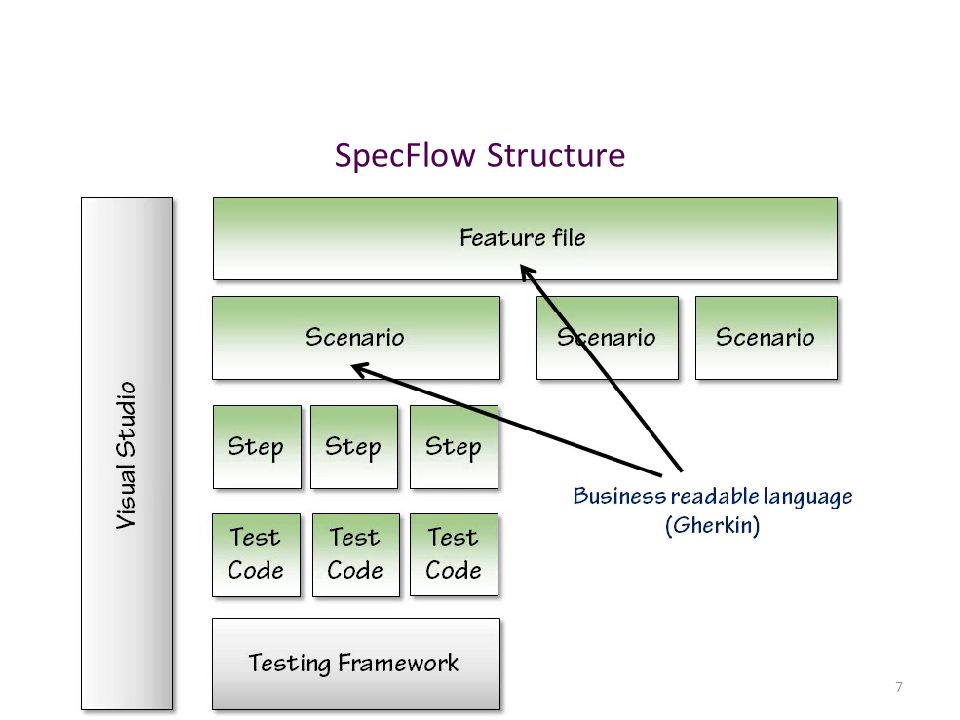
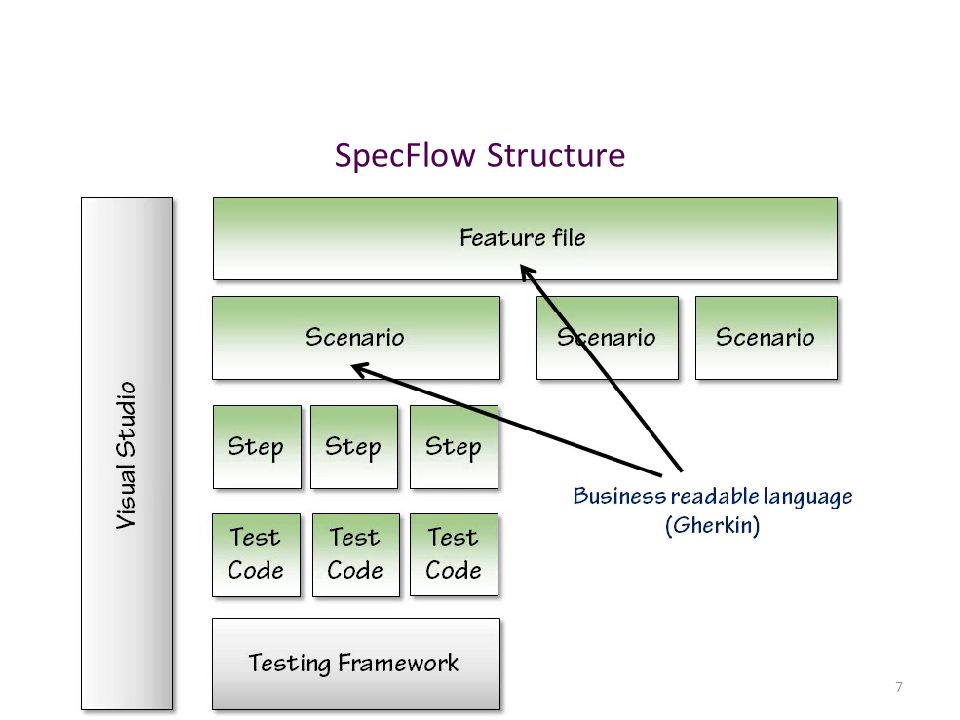
SpecFlow is inspired by Cucumber framework in the Ruby on Rails world. Cucumber uses plain English in the Gherkin format to express user stories. Once the user stories and their expectations are written, the Cucumber gem is used to execute those stores. SpecFlow brings the same concept to the .NET world and allows the developer to express the feature in plain English language. It also allows to write specification in human-readable Gherkin format.
Test-Driven Development
TDD is an iterative development process. Each iteration starts with a set of tests written for a new piece of functionality. These tests are supposed to fail during the start of iteration as there will be no application code corresponding to the tests. In the next phase of the iteration, Application code is written with an intention to pass all the tests written earlier in the iteration. Once the application code is ready tests are run.
Any failures in the test run are marked and more Application code is written/re-factored to make these tests pass. Once application code is added/re-factored the tests are run again. This cycle keeps on happening until all the tests pass. Once all the tests pass we can be sure that all the features for which tests were written have been developed.
Benefits of TDD
- Unit test proves that the code actually works
- Can drive the design of the program
- Refactoring allows improving the design of the code
- Low-Level regression test suite
- Test first reduce the cost of the bugs
- Developer can consider it as a waste of time
- The test can be targeted on verification of classes and methods and not on what the code really should do
- Test become part of the maintenance overhead of a project
- Rewrite the test when requirements change
Behavior Driven Development
- Tests are written in plain descriptive English type grammar
- Tests are explained as behavior of application and are more user-focused
- Using examples to clarify requirements.
This difference brings in the need to have a language that can define, in an understandable format.
Features of BDD
- Shifting from thinking in “tests” to thinking in “behavior”
- Collaboration between Business stakeholders, Business Analysts, QA Team and developers
- Ubiquitous language, it is easy to describe
- Driven by Business Value
- Extends Test-Driven Development (TDD) by utilizing natural language that non-technical stakeholders can understand